Remark :
“비동기(Asynchronous)”의 라틴어 어원은 다음과 같습니다:
- “Asyn”은 라틴어에서 “non” 또는 “not”을 의미하는 “a-” 접두사와 “together”를 의미하는 “syn-“의 결합입니다.
- “Chronous”는 라틴어 “chronus”에서 파생되었으며, “시간”을 의미합니다.
따라서 “Asynchronous”는 “시간적으로 동일하지 않는” 또는 “동시에 일어나지 않는”이라는 의미를 갖습니다.
Microsoft Visual Studio Community 2019
버전 16.9.3
The Task asynchronous programming model (TAP) provides an abstraction over asynchronous code
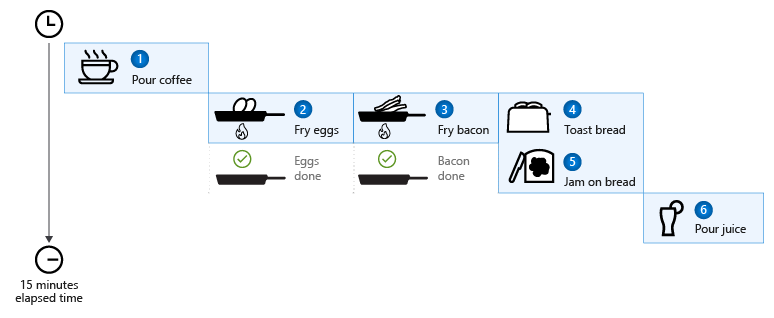
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 |
using Newtonsoft.Json; using System; using System.IO; using System.Net.Http; using System.Net.Http.Json; using System.Threading.Tasks; using System.Collections.Generic; namespace SystemNetHttpJsonSamples { partial class Program { static async Task Main(string[] args) { Coffee cup = PourCoffee(); Console.WriteLine("coffee is ready"); var eggsTask = FryEggsAsync(2); var baconTask = FryBaconAsync(3); var toastTask = MakeToastWithButterAndJamAsync(2); var breakfastTasks = new List<Task> { eggsTask, baconTask, toastTask }; while (breakfastTasks.Count > 0) { Task finishedTask = await Task.WhenAny(breakfastTasks); if (finishedTask == eggsTask) { Console.WriteLine("eggs are ready"); } else if (finishedTask == baconTask) { Console.WriteLine("bacon is ready"); } else if (finishedTask == toastTask) { Console.WriteLine("toast is ready"); } breakfastTasks.Remove(finishedTask); } Juice oj = PourOJ(); Console.WriteLine("oj is ready"); Console.WriteLine("Breakfast is ready!"); } static async Task<Toast> MakeToastWithButterAndJamAsync(int number) { var toast = await ToastBreadAsync(number); ApplyButter(toast); ApplyJam(toast); return toast; } private static Juice PourOJ() { Console.WriteLine("Pouring orange juice"); return new Juice(); } private static void ApplyJam(Toast toast) => Console.WriteLine("Putting jam on the toast"); private static void ApplyButter(Toast toast) => Console.WriteLine("Putting butter on the toast"); private static async Task<Toast> ToastBreadAsync(int slices) { for (int slice = 0; slice < slices; slice++) { Console.WriteLine("Putting a slice of bread in the toaster"); } Console.WriteLine("Start toasting..."); await Task.Delay(3000); Console.WriteLine("Remove toast from toaster"); return new Toast(); } private static async Task<Bacon> FryBaconAsync(int slices) { Console.WriteLine($"putting {slices} slices of bacon in the pan"); Console.WriteLine("cooking first side of bacon..."); await Task.Delay(3000); for (int slice = 0; slice < slices; slice++) { Console.WriteLine("flipping a slice of bacon"); } Console.WriteLine("cooking the second side of bacon..."); await Task.Delay(3000); Console.WriteLine("Put bacon on plate"); return new Bacon(); } private static async Task<Egg> FryEggsAsync(int howMany) { Console.WriteLine("Warming the egg pan..."); await Task.Delay(3000); Console.WriteLine($"cracking {howMany} eggs"); Console.WriteLine("cooking the eggs ..."); await Task.Delay(3000); Console.WriteLine("Put eggs on plate"); return new Egg(); } private static Coffee PourCoffee() { Console.WriteLine("Pouring coffee"); return new Coffee(); } private class Coffee { } private class Egg { } private class Bacon { public Bacon() { } } private class Toast { public Toast() { } } private class Juice { public Juice() { } } } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
Pouring coffee coffee is ready Warming the egg pan... putting 3 slices of bacon in the pan cooking first side of bacon... Putting a slice of bread in the toaster Putting a slice of bread in the toaster Start toasting... flipping a slice of bacon flipping a slice of bacon flipping a slice of bacon cooking the second side of bacon... cracking 2 eggs cooking the eggs ... Remove toast from toaster Putting butter on the toast Putting jam on the toast toast is ready Put bacon on plate Put eggs on plate bacon is ready eggs are ready Pouring orange juice oj is ready Breakfast is ready! |